Here's my flash configuration.
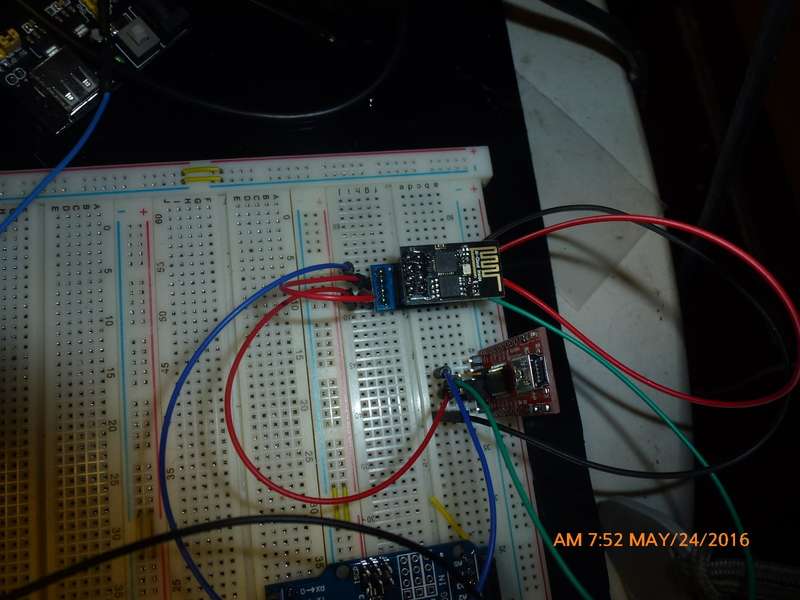
I know, hard to see how it's wired, but I'm able to flash it properly using this:
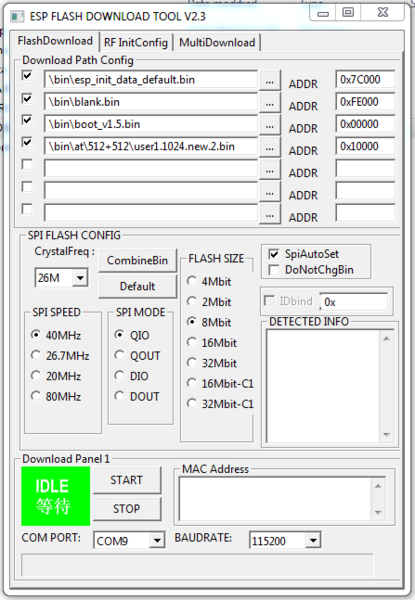
The path's to the files aren't complete because I wanted to show which files are being used. I got the flasher program and the BIN files this morning.
This is it wired into the UNO.
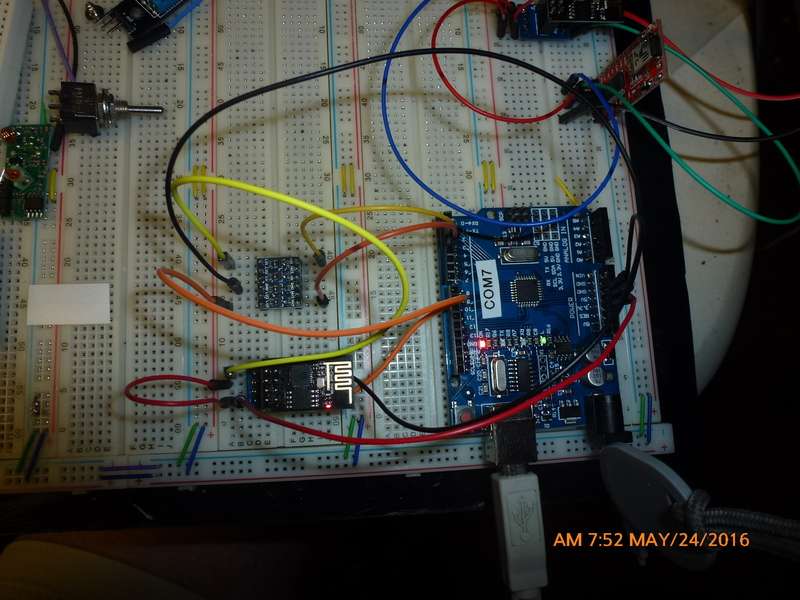
It's going through a level shifter on the TX/RX pins.
This is the sketch I'm using. Yes, I did remove the SSID, Pass and Webserver IP, but they're correct in my version here.
#include <SoftwareSerial.h>
#include <stdlib.h>
// RX ESP -> TX Arduino
// TX ESP -> RX Arduino
SoftwareSerial ESPSerial(2,3); // RX, TX
// this runs once
void setup() {
// enable debug serial
Serial.begin(9600);
// enable software serial
ESPSerial.begin(9600);
while(!ESPSerial){
;
}
// reset ESP8266
ESPSerial.println("AT+RST");
//conect to network
ESPSerial.println("AT+CWMODE=1");
ESPSerial.println("AT+CWJAP=\"<ssid>\",\"<pass>\"");
delay(8000);
}
// the loop
void loop() {
// TCP connection
String cmd = "AT+CIPSTART=\"TCP,\"<webserverIP>\",\"80\"";
Serial.println(cmd);
ESPSerial.println(cmd);
if(ESPSerial.find("Error")){
Serial.println("AT+CIPSTART error");
return;
}
// prepare GET string to send - page to get - val.php
String getStr = "GET /test.php?string=";
// String to display
getStr += "fromESPv4";
getStr += "\r\n\r\n";
Serial.println(getStr);
// send data length
cmd = "AT+CIPSEND=";
cmd += String(getStr.length());
ESPSerial.println(cmd);
Serial.println(cmd);
if(ESPSerial.find(">")){
ESPSerial.println(getStr);
}
else{
ESPSerial.println("AT+CIPCLOSE");
// alert user
Serial.println("AT+CIPCLOSE");
}
delay(1000);
}
I don't think this is part of the problem, but here's the PHP script using just to test things out. My script does work just doing it manually, so it's definitely something in the communication aspect, not the PHP script.
<?php
$val = $_GET['string'];
$bf = fopen("test.txt","w+");
fwrite ($bf,$val);
fclose($bf);
?>
Suggestions on what I"m doing wrong? Right now I'm not seeing any kind of serial activity (via the blinky blue LED) on the ESP8266