To make the code work well, you must stop the winsock connection, otherwise it is too slow. I've not looked at how to end it on program run. Just hit the back arrow on your browser to get back to the edit screen. You can see the rotary encoder incrementing up and down through either the serial port or start the UDP viewer shown in the following thread:
http://www.esp8266.com/viewtopic.php?f=40&t=9451&hilit=udp
Don't forget to change the UDP address it broadcasts to match your subnet!
Here is the code:
'******************************************************
'* Rotary encoder with switch *
'* by Russ McIntire, 7/4/16 *
'* Stolen heavily from: *
'* http://bildr.org/2012/08/rotary-encoder-arduino/ *
'* D4 for switch *
'* D2 for LSB rotary *
'* D3 for MSB rotary *
'******************************************************
udpbegin 5001
msb = 0 'D3 rotary bit
lsb = 0 'D2 rotary bit
encoded = 0 'Combined MSB and LSB
lastencoded = 3 'Previous MSB and LSB - set to 3 to allow the first calculation to work
sum = 0 'Place lastencoded in front of encoded
encoder = 0 'encoder value
'Listen for rotary encoder input
interrupt D4, [switch]
interrupt D2, [change]
interrupt D3, [change]
wait
end
[switch]
if io(laststat,D4) = 1 then
serialprintln "switch high"
udpwrite "192.168.10.255", 5001, "SH"
else
serialprintln "switch low"
udpwrite "192.168.10.255", 5001, "SL"
endif
wait
[change]
msb = io(laststat,D2)
lsb = io(laststat,D3)
encoded = lsb + (msb << 1) 'Combine MSB and LSB
sum = lastencoded << 2 + encoded 'Put lastencoded in the front and call it sum
lastencoded = encoded 'record lastencoded
if (sum = 13 or sum = 4 or sum = 2 or sum = 11) then
encoder = encoder - 1
endif
if (sum = 14 or sum = 7 or sum = 1 or sum = 8) then
encoder = encoder + 1
endif
'serialprintln " "
'serialprintln "msb = "&msb
'serialprintln "lsb = "&lsb
'serialprintln "sum = "&sum
serialprintln "encoder = "&encoder
udpwrite "192.168.10.255", 5001, "E"&str(encoder)
wait
Here is a picture of the perfboard layout, the resistors are 10K.
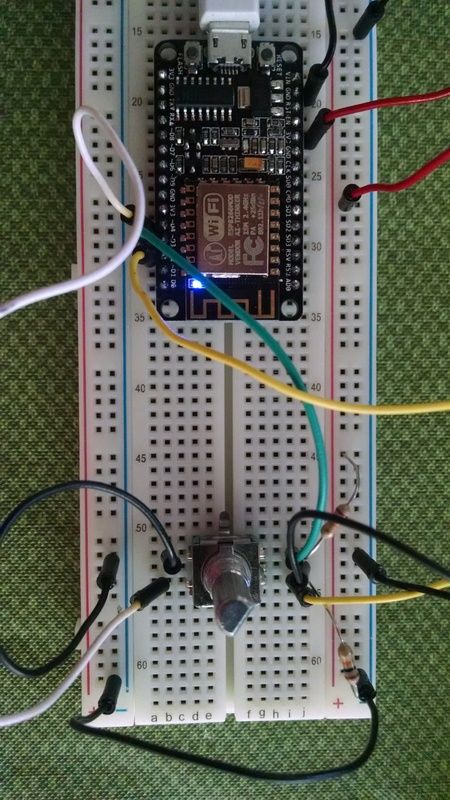
The rotary encoder is the cheap ones from electrodragon. You can purchase one with the resistors already mounted on a pcb suitable to plug into your breadboard as well. Additional info on connecting the rotary encoder can be found here:
http://henrysbench.capnfatz.com/henrys-bench/arduino-sensors-and-input/keyes-ky-040-arduino-rotary-encoder-user-manual/
Within the code in the header is a link to where I stole heavily in writing my basic code. Enjoy, hopefully you can use the ideas in a project. I'd love to hear back on:
1) how to turn off winsock programmatically
2) make a change to reduce the impact on the ESP maintaining the winsock connection to improve performance
3) tweak my setup to make it run a little more smoothly. Sometimes it really jumps about, the encoder I'm using gives 4 steps for each detente. I've used rotary encoders in other projects on PIC HW, here's a link:
http://cabcommand.com/