I purchased a NodeMCU, and an Arduino starter kit to play with over my Christmas break and I'm trying to use the NodeMCU with an Ultrasonic Sensor(HC-SR04) but for some reason the sensor always reads zero for distance(when hooked up to the Arduino it reads properly so I know its not busted). I am fairly new to all of this but neither my own code nor the code from the guide I used to test worked. I have attached an image of my setup but just to clarify I have
VCC > 5V(Power supply)
GND > GND
Trig > D1
Echo > D2
The NodeMCU(Amica, actually a V2 board not V1 like the image) is being powered via USB to my Macbook and the Power Supply is powered via wall plugin.
I have 5V coming out of the power supply as I've tested it with my multimeter.(have also tested with a 3.3V supply as recommended online)
My code is as follows
// defines pins numbers
const int trigPin = 5; //D1
const int echoPin = 4; //D2
// defines variables
long duration;
int distance;
void setup() {
pinMode(trigPin, OUTPUT); // Sets the trigPin as an Output
pinMode(echoPin, INPUT); // Sets the echoPin as an Input
Serial.begin(9600); // Starts the serial communication
}
void loop() {
// Clears the trigPin
digitalWrite(trigPin, LOW);
delayMicroseconds(2);
// Sets the trigPin on HIGH state for 10 micro seconds
digitalWrite(trigPin, HIGH);
delayMicroseconds(10);
digitalWrite(trigPin, LOW);
// Reads the echoPin, returns the sound wave travel time in microseconds
duration = pulseIn(echoPin, HIGH);
// Calculating the distance
distance= duration*0.034/2;
// Prints the distance on the Serial Monitor
Serial.print("Distance: ");
Serial.println(distance);
delay(2000);
}
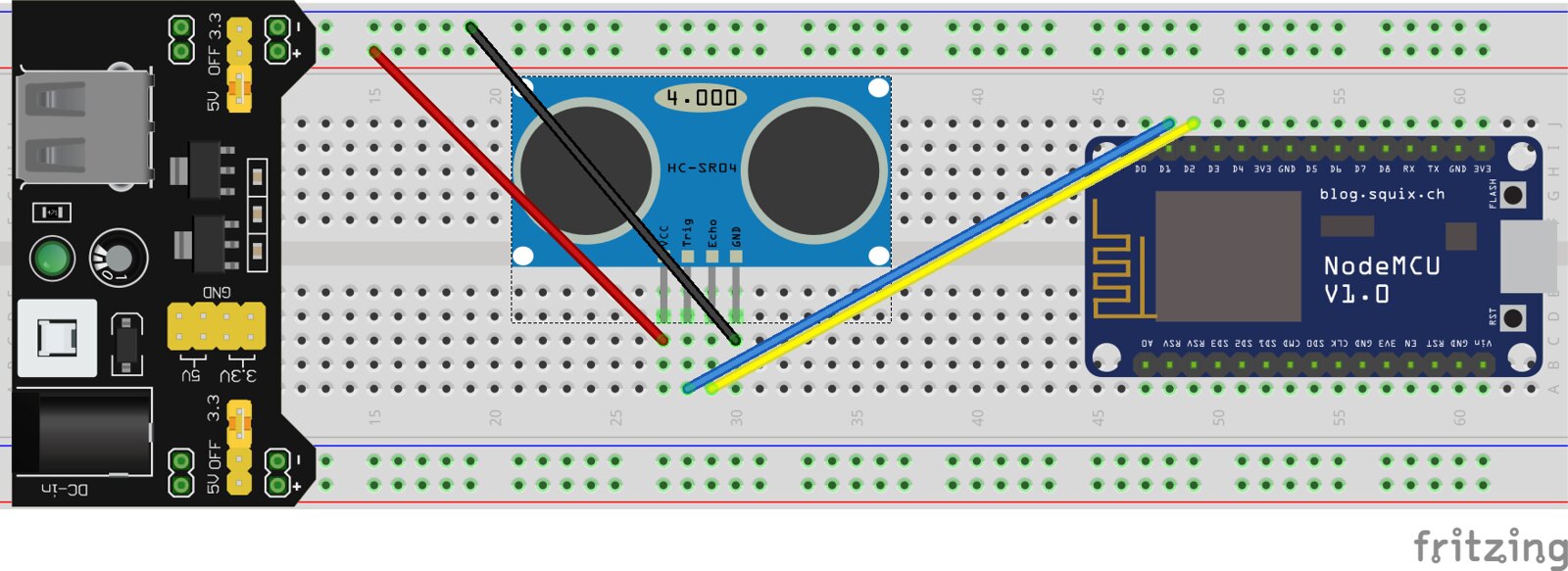
If you have ever had this issue or see something glaringly wrong with my code please let me know! I can't seem to solve why it reads zero through my NodeMCU and correctly on my Uno.