For smartconfig, I have used and tested it. I used app from this github repository IOT-Espressif-Android and use espressif iot SDK v1.1.1. They work perfectly.
Unfortunately, adding any code from esp_mqtt cause the firmware to not working anymore. I am still looking on how to use smartconfig and esp_mqtt together. I attached my code:
/******************************************************************************
* Copyright 2013-2014 Espressif Systems (Wuxi)
*
* FileName: user_main.c
*
* Description: entry file of user application
*
* Modification history:
* 2014/1/1, v1.0 create this file.
*******************************************************************************/
#include "ets_sys.h"
#include "osapi.h"
#include "driver/uart.h"
#include "smartconfig.h"
#include "user_interface.h"
#include "mqtt.h"
#include "wifi.h"
#include "config.h"
#include "debug.h"
#include "gpio.h"
#include "user_interface.h"
#include "mem.h"
sc_type SC_Type = 0;
MQTT_Client mqttClient;
void wifiConnectCb(uint8_t status)
{
if(status == STATION_GOT_IP){
MQTT_Connect(&mqttClient);
} else {
MQTT_Disconnect(&mqttClient);
}
}
void mqttConnectedCb(uint32_t *args)
{
MQTT_Client* client = (MQTT_Client*)args;
INFO("MQTT: Connected\r\n");
MQTT_Subscribe(client, "/mqtt/topic/0", 0);
MQTT_Subscribe(client, "/mqtt/topic/1", 1);
MQTT_Subscribe(client, "/mqtt/topic/2", 2);
MQTT_Publish(client, "/mqtt/topic/0", "hello0", 6, 0, 0);
MQTT_Publish(client, "/mqtt/topic/1", "hello1", 6, 1, 0);
MQTT_Publish(client, "/mqtt/topic/2", "hello2", 6, 2, 0);
}
void mqttDisconnectedCb(uint32_t *args)
{
MQTT_Client* client = (MQTT_Client*)args;
INFO("MQTT: Disconnected\r\n");
}
void mqttPublishedCb(uint32_t *args)
{
MQTT_Client* client = (MQTT_Client*)args;
INFO("MQTT: Published\r\n");
}
void mqttDataCb(uint32_t *args, const char* topic, uint32_t topic_len, const char *data, uint32_t data_len)
{
char *topicBuf = (char*)os_zalloc(topic_len+1),
*dataBuf = (char*)os_zalloc(data_len+1);
MQTT_Client* client = (MQTT_Client*)args;
os_memcpy(topicBuf, topic, topic_len);
topicBuf[topic_len] = 0;
os_memcpy(dataBuf, data, data_len);
dataBuf[data_len] = 0;
INFO("Receive topic: %s, data: %s \r\n", topicBuf, dataBuf);
os_free(topicBuf);
os_free(dataBuf);
}
void ICACHE_FLASH_ATTR
smartconfig_done(sc_status status, void *pdata)
{
switch(status) {
case SC_STATUS_WAIT:
os_printf("SC_STATUS_WAIT\n");
break;
case SC_STATUS_FIND_CHANNEL:
os_printf("SC_STATUS_FIND_CHANNEL\n");
break;
case SC_STATUS_GETTING_SSID_PSWD:
os_printf("SC_STATUS_GETTING_SSID_PSWD\n");
break;
case SC_STATUS_LINK:
os_printf("SC_STATUS_LINK\n");
struct station_config *sta_conf = pdata;
wifi_station_set_config(sta_conf);
wifi_station_disconnect();
wifi_station_connect();
break;
case SC_STATUS_LINK_OVER:
os_printf("SC_STATUS_LINK_OVER\n");
if (SC_Type == SC_TYPE_ESPTOUCH) {
uint8 phone_ip[4] = {0};
os_memcpy(phone_ip, (uint8*)pdata, 4);
os_printf("Phone ip: %d.%d.%d.%d\n",phone_ip[0],phone_ip[1],phone_ip[2],phone_ip[3]);
}
smartconfig_stop();
break;
}
}
void user_rf_pre_init(void)
{
}
void user_init(void)
{
uart_init(BIT_RATE_115200, BIT_RATE_115200);
os_delay_us(1000000);
os_printf("SDK version:%s\n", system_get_sdk_version());
SC_Type = SC_TYPE_ESPTOUCH;
wifi_set_opmode(STATION_MODE);
smartconfig_start(SC_Type, smartconfig_done);
CFG_Load();
MQTT_InitConnection(&mqttClient, sysCfg.mqtt_host, sysCfg.mqtt_port, sysCfg.security);
//MQTT_InitConnection(&mqttClient, "192.168.11.122", 1880, 0);
MQTT_InitClient(&mqttClient, sysCfg.device_id, sysCfg.mqtt_user, sysCfg.mqtt_pass, sysCfg.mqtt_keepalive, 1);
//MQTT_InitClient(&mqttClient, "client_id", "user", "pass", 120, 1);
MQTT_InitLWT(&mqttClient, "/lwt", "offline", 0, 0);
MQTT_OnConnected(&mqttClient, mqttConnectedCb);
MQTT_OnDisconnected(&mqttClient, mqttDisconnectedCb);
MQTT_OnPublished(&mqttClient, mqttPublishedCb);
MQTT_OnData(&mqttClient, mqttDataCb);
WIFI_Connect(sysCfg.sta_ssid, sysCfg.sta_pwd, wifiConnectCb);
INFO("\r\nSystem started ...\r\n");
}
Here is my correct smartconfig output:
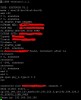
And this is what happen when I add esp_mqtt code:
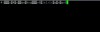
Now, I think this is because in the SDK docs, it states that smartconfig_start must run alone, we cannot run other code with it. My question is, how can I run other function in ESP8266 after smartconfig finished?