For that I need to control a stepper motor with a ESP-12. I want to create it in such a way that I can trigger the servo with a http call.
To connect the stepper motor to the ESP-12 I used a A3967 EasyDriver module and a LM1117 to convert the 5v coming out of the EasyDriver to 3.3v to power the ESP.
I hooked it up much like this, except for a arduino I used the ESP with pins 4 and 5:
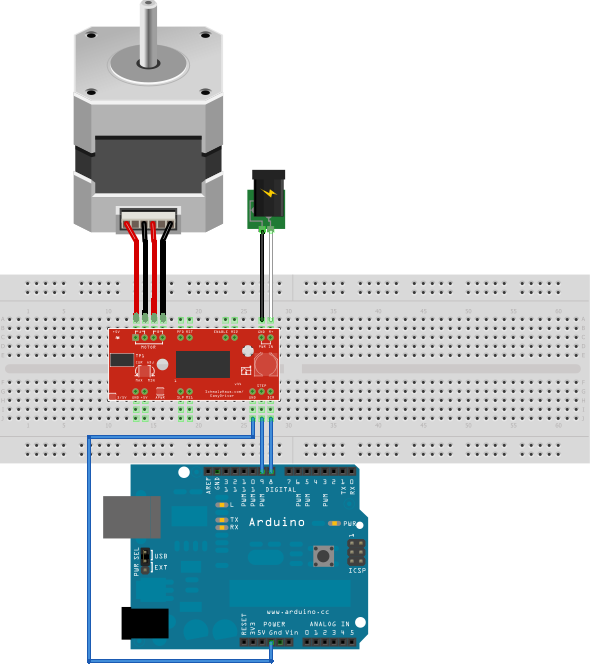
When I upload a very basic sketch to make the stepper motor spin, it works like a charm:
#include <AccelStepper.h>
// Define a stepper and the pins it will use
AccelStepper stepper(1, 5, 4);
int pos = 6600;
void setup()
{
stepper.setMaxSpeed(10000);
stepper.setAcceleration(1000);
}
void loop()
{
if (stepper.distanceToGo() == 0)
{
delay(500);
pos = -pos;
stepper.moveTo(pos);
}
stepper.run();
}
Now I want to do the same upon a http request, so I modified the code like this:
#include <AccelStepper.h>
#include <ESP8266WiFi.h>
#include <WiFiClient.h>
#include <ESP8266WebServer.h>
#include <ESP8266mDNS.h>
// Define a stepper and the pins it will use
AccelStepper stepper(1, 5, 4);
int pos = 6600;
const char* ssid = "hes";
const char* password = "somepw";
ESP8266WebServer server(80);
const int led = 13;
void handleRoot() {
stepper.moveTo(6600);
stepper.run();
server.send(200, "text/plain", "running stepper!");
}
void setup(void){
stepper.setMaxSpeed(10000);
stepper.setAcceleration(1000);
Serial.begin(115200);
WiFi.begin(ssid, password);
Serial.println("");
// Wait for connection
while (WiFi.status() != WL_CONNECTED) {
delay(500);
Serial.print(".");
}
Serial.println("");
Serial.print("Connected to ");
Serial.println(ssid);
Serial.print("IP address: ");
Serial.println(WiFi.localIP());
server.on("/", handleRoot);
server.begin();
Serial.println("HTTP server started");
}
void loop(void){
server.handleClient();
}
Problem is that the HTTP server runs fine, but the stepper motor doesn't run. I feel it twitch a little bit when I do the HTTP request, but it doesn't spin like it should.
When I put the commands
stepper.moveTo(6600);
stepper.run();
inside the loop() function, it does make a spin upon boot.
Any idea where I'm going wrong?